上篇文章咱们讲到了怎么配置服务的DataSource数据库数据源,方便其对数据库进行操作.这篇文章主要讲解一下咱们运用整合的JDBC进行简略的数据添加操作.
创立用于做测验数据的模拟数据表
```sql
USE test;
CREATE TABLE article (
id INT(10) AUTO_INCREMENT,
title VARCHAR(100) NOT NULL,
description VARCHAR(200),
PRIMARY KEY (id)
);
```
创立数据表对应实体类(在源码目录下新建bean文件夹, 并在该文件夹下创立相应的Article类文件)
```java
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edurt.bean;
/**
* Article <br/>
* 描绘 : Article <br/>
* 作者 : qianmoQ <br/>
* 版别 : 1.0 <br/>
* 创立时间 : 2018-03-26 下午4:51 <br/>
* 联络作者 : <a href="mailTo:shichengoooo@163.com">qianmoQ</a>
*/
public class Article {
private Integer id;
private String title;
private String description;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
@Override
public String toString() {
return "Article{" +
"id=" + id +
", title='" + title + '\'' +
", description='" + description + '\'' +
'}';
}
}
```
创立数据表底层数据库操作Repository(在源码目录下创立repository文件夹, 并在该目录下创立ArticleRepository类文件)
```java
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edurt.repository;
import com.edurt.bean.Article;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Component;
/**
* ArticleRepository <br/>
* 描绘 : ArticleRepository <br/>
* 作者 : qianmoQ <br/>
* 版别 : 1.0 <br/>
* 创立时间 : 2018-03-26 下午4:54 <br/>
* 联络作者 : <a href="mailTo:shichengoooo@163.com">qianmoQ</a>
*/
@Component
public class ArticleRepository {
@Autowired
private JdbcTemplate jdbcTemplate; // 引进数据库操作模型
/**
* 创立文章信息
*
* @param article 文章信息
*/
public void createArticle(Article article) {
String sql = "INSERT INTO article(title, description) VALUES(?, ?)";
jdbcTemplate.update(sql, article.getTitle(), article.getDescription());
}
}
```
创立数据库业务层Service (在源码目录下创立service文件夹, 并在该目录下创立ArticleService)
```java
package com.edurt.service;
import com.edurt.bean.Article;
public interface ArticleService {
void createArticle(Article article);
}
```
创立接口实现ArticleServiceImpl类文件
```java
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edurt.service;
import com.edurt.bean.Article;
import com.edurt.repository.ArticleRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* ArticleServiceImpl <br/>
* 描绘 : ArticleServiceImpl <br/>
* 作者 : qianmoQ <br/>
* 版别 : 1.0 <br/>
* 创立时间 : 2018-03-26 下午5:01 <br/>
* 联络作者 : <a href="mailTo:shichengoooo@163.com">qianmoQ</a>
*/
@Service(value = "articleService")
public class ArticleServiceImpl implements ArticleService {
@Autowired
private ArticleRepository articleRepository;
@Override
public void createArticle(Article article) {
articleRepository.createArticle(article);
}
}
```
创立API接口层Controller (在源码目录下创立controller文件夹, 并在该目录下创立ArticleController类文件)
```java
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
* <p>
* http://www.apache.org/licenses/LICENSE-2.0
* <p>
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.edurt.controller;
import com.edurt.bean.Article;
import com.edurt.service.ArticleService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
/**
* ArticleController <br/>
* 描绘 : ArticleController <br/>
* 作者 : qianmoQ <br/>
* 版别 : 1.0 <br/>
* 创立时间 : 2018-03-26 下午5:08 <br/>
* 联络作者 : <a href="mailTo:shichengoooo@163.com">qianmoQ</a>
*/
@RestController
@RequestMapping(value = "article")
public class ArticleController {
@Autowired
private ArticleService articleService;
@RequestMapping(value = "create", method = RequestMethod.POST)
String create(@RequestBody Article article) {
articleService.createArticle(article);
return "SUCCESS";
}
}
```
运用RestAPI测验东西测验添加数据我用的是PostMan软件
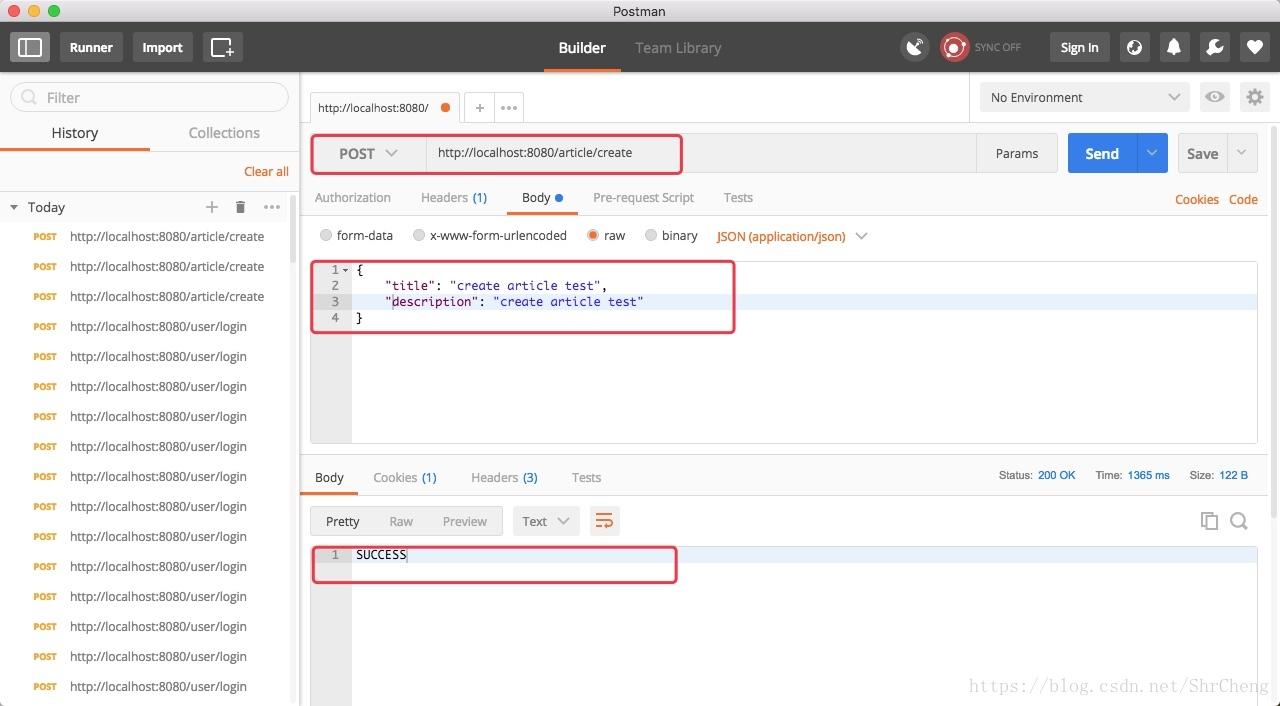
检查数据表是否已经写入该数据
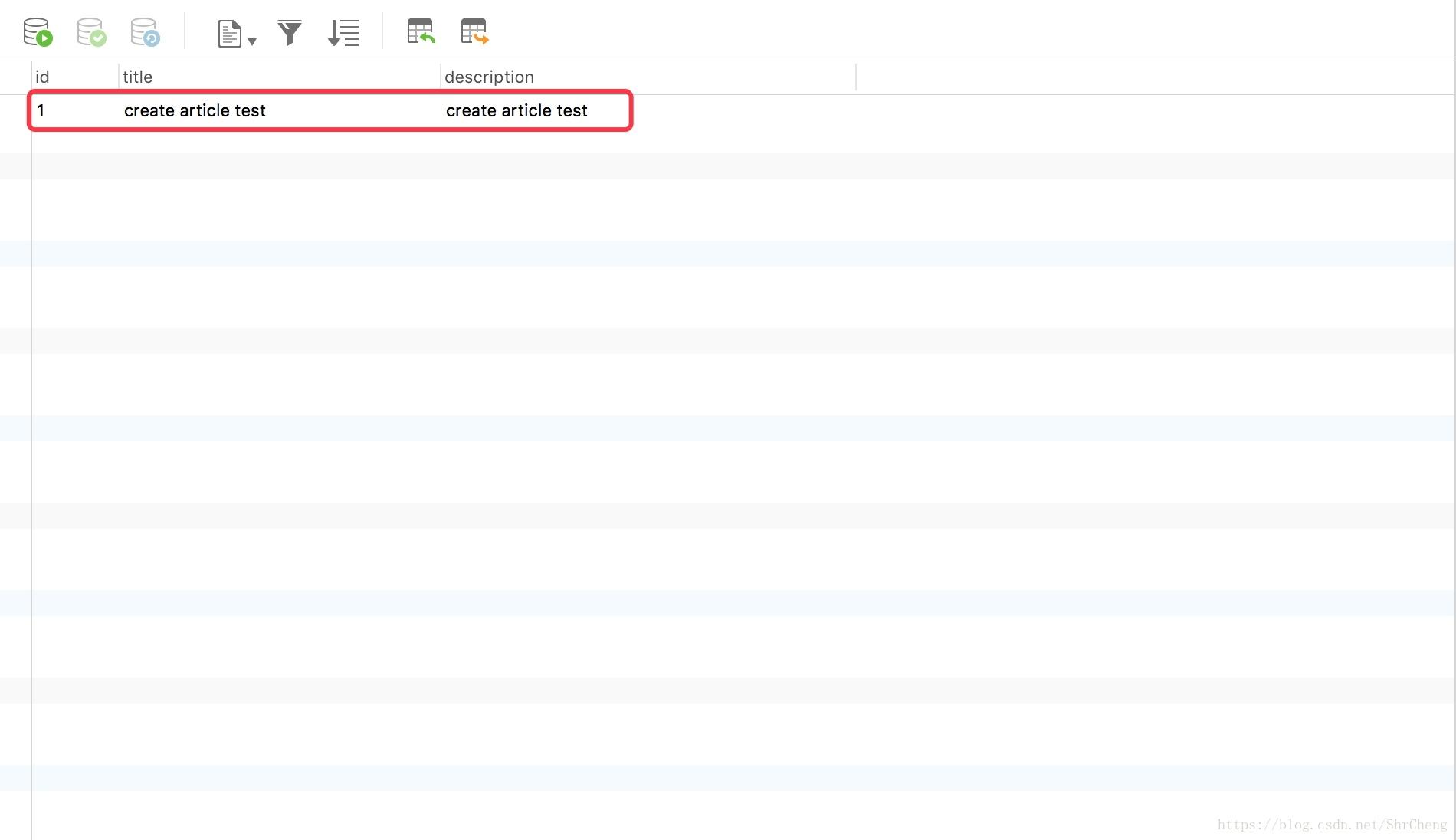
声明:本站所有文章,如无特殊说明或标注,均为本站原创发布。任何个人或组织,在未征得本站同意时,禁止复制、盗用、采集、发布本站内容到任何网站、书籍等各类媒体平台。如若本站内容侵犯了原著者的合法权益,可联系我们进行处理。